Introduction
When building dynamic websites in Webflow, it’s common to use CMS lists to display various types of content. But what happens when one of your CMS lists is empty? Instead of showing an empty section with a blank message or placeholder, it’s better to hide the entire section altogether.
This is particularly useful for use cases like author pages, where sections such as “Articles,” “Guides,” or “News” might not have any content for some authors. By hiding empty sections, you provide a cleaner, more professional user experience while maintaining the focus on relevant content.
In this guide, we’ll explore how to implement this functionality using the powerful :has
CSS selector and provide insights into how this was done using JavaScript before CSS :has
became widely supported.
What Is an Empty CMS Section?
An empty CMS section is a section on your Webflow site containing a CMS collection list with no items to display. In Webflow, this is indicated by the .w-dyn-empty
class automatically added to CMS lists that are empty.
For example, if you have sections for “Articles,” “News,” and “Guides,” some sections may not contain any items for specific authors. Instead of showing a placeholder or blank section, you can hide those sections dynamically.
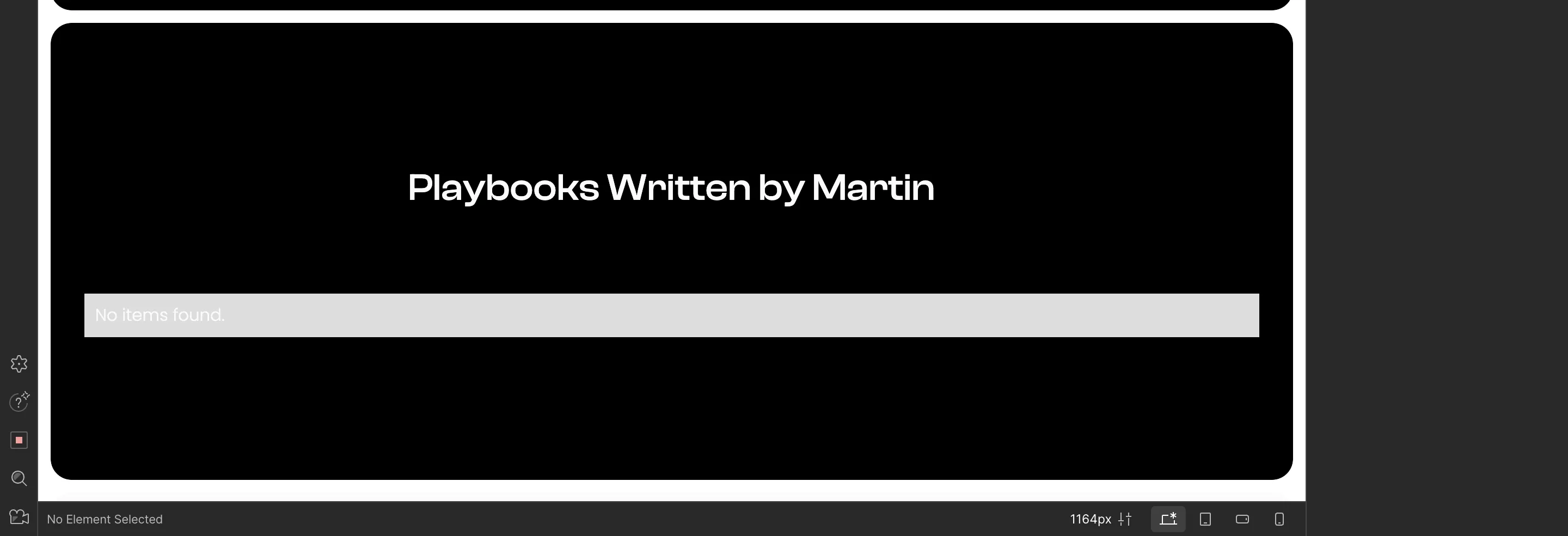
Example Use Case
Imagine you’re building an author page for a blog, and each author has the following content types:
- Articles
- News
- Guides
- Playbooks
Not all authors contribute to every type of content. By implementing the CSS method detailed below, only sections with actual content will be visible on the author’s page, ensuring a clean and streamlined design.
How to Hide Empty CMS Sections Using CSS
The simplest and most efficient way to hide sections with empty CMS lists is to use the :has
CSS selector. This approach leverages CSS’s native capabilities, ensuring cleaner code and better performance.
Preparation Steps
Before adding the CSS, ensure your sections are properly set up to be targeted:
- Go to the Section Element: Navigate to the section containing your CMS list.
- Open the Settings Panel: Click
D
on your keyboard to open the settings panel for the section. To find other ways to speed up your workflow, read our guide on Webflow shortcuts. - Add an Attribute: Scroll to the attributes section and add an attribute with the name
data-content-type
and the valueCMS
.
CSS Implementation
Add the following snippet to your Webflow site’s global custom code settings:
1<style>
2 section[data-content-type="CMS"]:has(.w-dyn-empty) {
3 display: none;
4 }
5</style>

How It Works
- The
section[data-content-type="CMS"]
selector targets all sections designated for CMS content. - The
:has(.w-dyn-empty)
selector checks if the section contains an element with the.w-dyn-empty class.
- If the
.w-dyn-empty
class is found, thedisplay: none;
rule hides the entire section.
Alternative CSS Method
Here’s another CSS method to hide sections with empty CMS lists. This approach checks if the section contains no .cms-item
elements:
<style>
section[data-content-type="CMS"]:not(:has(.cms-item)) {
display: none;
}
</style>
This alternative is particularly useful if you’re targeting CMS items directly rather than relying on the .w-dyn-empty
class.
Another benefit is that this method has a better experience when the code is embedded somewhere mid-page.
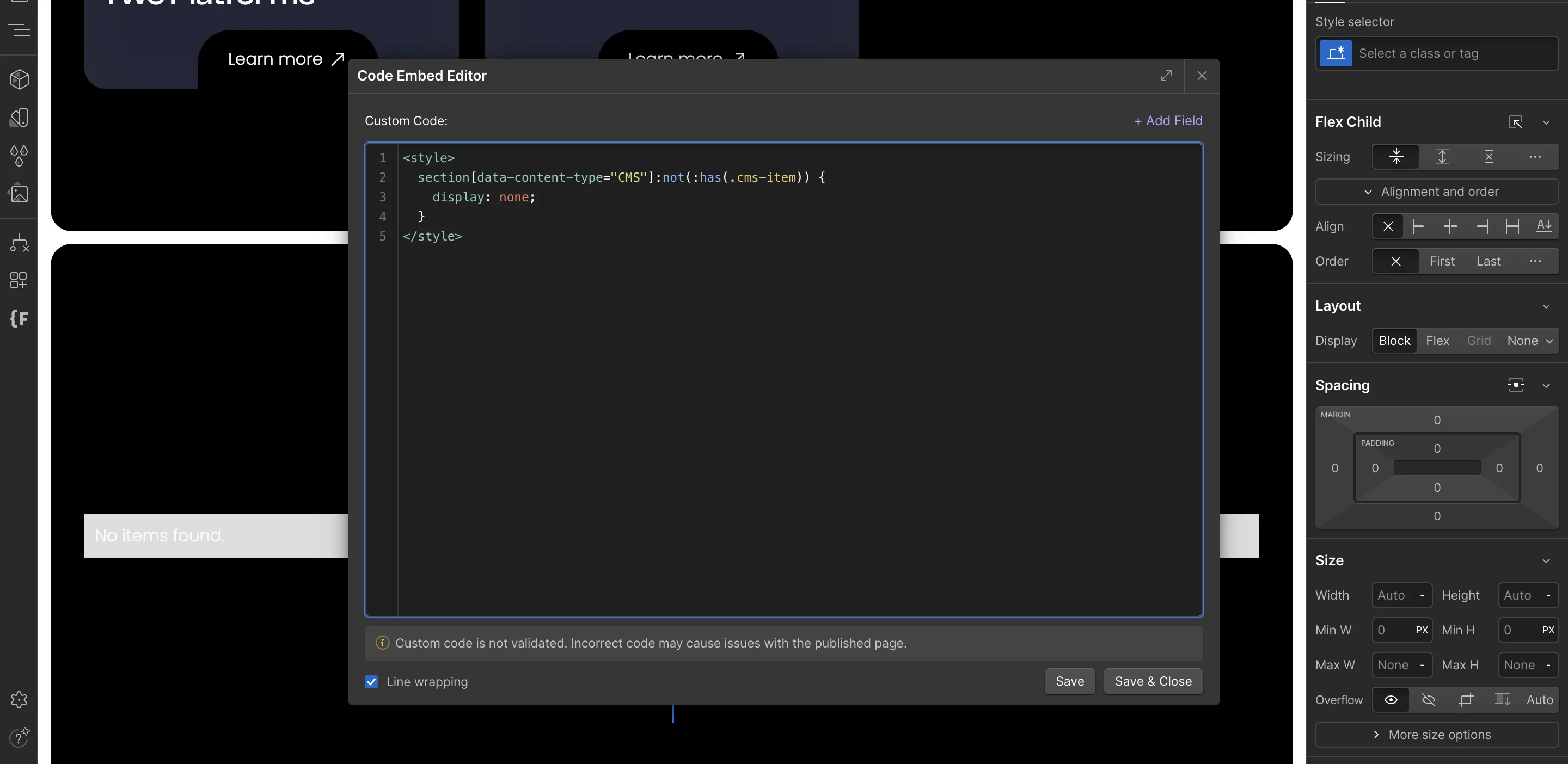
JavaScript: How It Was Done Before :has
Before the :has
selector was widely supported, developers relied on JavaScript to hide empty CMS sections. Here’s an example of how this functionality was previously implemented:
JavaScript Code Example
1document.addEventListener("DOMContentLoaded", function () {
2 // Select all sections with CMS content
3 const cmsSections = document.querySelectorAll('section[data-content-type="CMS"]');
4
5 cmsSections.forEach((section) => {
6 const cmsList = section.querySelector(".w-dyn-list");
7
8 // Check if the CMS list has the class indicating it's empty
9 if (cmsList && cmsList.querySelector(".w-dyn-empty")) {
10 section.style.display = "none"; // Hide the section if empty
11 }
12 });
13});
How It Works
- The script waits for the page to fully load using
DOMContentLoaded
. - It selects all CMS sections using the attribute
data-content-type="CMS"
. - For each section, it checks if the
.w-dyn-empty
class exists within the CMS list. - If found, the section’s
display
property is set tonone
, effectively hiding it.
Why JavaScript Was Necessary
In the past, CSS lacked the ability to select parent elements based on child conditions. JavaScript provided a way to traverse the DOM and apply conditional logic, albeit at the cost of increased complexity and potential performance issues.
Why CSS :has
Is the Preferred Solution
With the widespread support of the :has
selector, CSS has become the go-to solution for hiding empty CMS sections. Here’s why:
- Performance: CSS is handled by the browser’s rendering engine, making it faster and more efficient than JavaScript.
- Simplicity: The CSS solution is more concise and easier to maintain.
- Browser Support: The
:has
selector is now supported by most modern browsers, eliminating the need for JavaScript in many scenarios.
Conclusion
Hiding sections with empty CMS lists is a crucial step in creating professional, user-friendly websites. By leveraging CSS :has
, you can achieve this functionality with minimal effort and maximum efficiency. If you find implementing this solution challenging, our Webflow Development Agency can provide expert assistance to help you optimize your project. For those curious about how this was managed before, the JavaScript example demonstrates the evolution of web development techniques.
Whether you’re building a blog, author page, or any other CMS-driven site, hiding empty sections ensures a cleaner design and better user experience. Give it a try and take your Webflow projects to the next level!