Text animations can elevate your web design, turning simple phrases into eye-catching visuals. Whether it's for a hero header or a call-to-action, dynamic text can engage users and leave a lasting impression. This guide introduces SplitType, a JavaScript library that makes it easy to split text into characters, words, or lines for creative animations.
In this tutorial, you’ll learn how to use SplitType in a Webflow project to create animations for text that fits within a single line.
What Is SplitType?
SplitType is a lightweight JavaScript library that divides text into manageable elements, such as characters, words, or lines. This allows you to animate each element individually, opening the door for creative and precise animations.
It is a well known text splitting library used for award winning animations.

Benefits of SplitType
- Fine-Grained Control: Target individual characters, words, or lines for animation.
- Lightweight: Easy to integrate without bloating your project.
- Versatile: Works well with CSS and JavaScript animations.
Drawbacks of SplitType
While SplitType is a powerful tool for creating text animations, it has its drawbacks:
- Performance Considerations: For static text, manually wrapping characters in
<span>
tags within the HTML can be more efficient than using a library. This reduces the overhead introduced by JavaScript processing. - Complexity for Multi-Line Text: While SplitType supports multi-line text, implementing animations for these requires aditional coding if you're focused on performance, or the use of aditional libraries that can make your site heavier.
- Dependence on JavaScript: If JavaScript is disabled in the browser, the animations will not work.
When to Use SplitType
- Hero Sections: Create an impactful introduction to your site.
- Call-to-Actions: Draw attention to key interactive elements.
- Headers and Titles: Add personality to static text.
Prerequisites
To get started, ensure you have the following:
- Webflow Project: Custom code enabled (any paid plan).
- Basic CSS and JavaScript Knowledge: For styling and functionality.
- SplitType Library: Available via a CDN link.
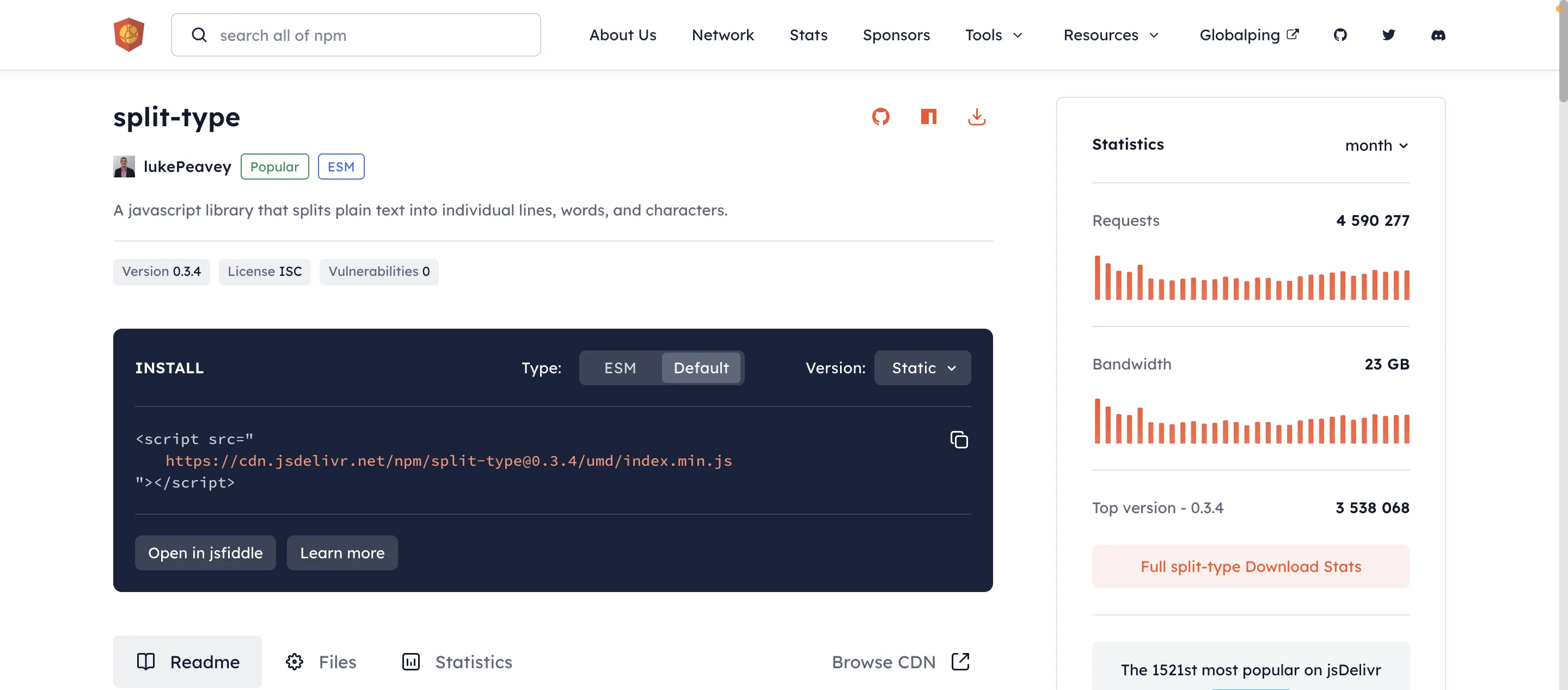
Step-by-Step Implementation
Step 1: Add the SplitType Library
Include the SplitType library in your Webflow project. Go to Page Settings > Custom Code, and add the following script in the <head>
or before the closing <body>
tag:
1<script src="https://cdn.jsdelivr.net/npm/split-type@0.3.4/umd/index.min.js"></script>
Step 2: Markup Your Text
Wrap the text you want to animate with a container and assign it the class .split-anim
:
1<div class="split-anim">Your Award-Winning Text Animation</div>
Step 3: Add CSS for Animation
In your Webflow Custom Code settings, paste the following CSS:
1:root{--i: 0; --j: 0;}
2.split-anim .word .char {transform: translateY(110%);}
3.split-anim.is-active .char {
4 animation: split-anim 0.15s ease-out forwards;
5 animation-delay: calc(var(--j) * 0.3s + var(--i) * 0.05s);
6}
7@keyframes split-anim{
8 0%{transform: rotate(-25deg) translateY(110%);}
9 100% {transform: rotate(0deg) translateY(0%);}
10}
Keep in mind that you have to calculate an animation delay based on variables to ensure that the text is staggered. Each item must correspond with a variable's number.

Ensure that .word
has overflow: hidden;
set to it's css.
Step 4: Write JavaScript for SplitType and Animation
Add the following script to detect when the text enters the viewport and activate the animation:
1// Initialize
2SplitTypelet text = new SplitType('.split-anim', { types: 'chars' });
3// Helper function to check if an element is in the viewport
4function isInViewport(element) {
5 let rect = element.getBoundingClientRect();
6 return rect.top >= 0 && rect.top <= window.innerHeight;
7}
8// Add .is-active class when elements enter the viewport
9function activateSplit() {
10 document.querySelectorAll('.split-anim').forEach(element => {
11 if (isInViewport(element)) {
12 element.classList.add('is-active');
13 }
14 });
15 }
16// Attach scroll event listener
17window.addEventListener('scroll', activateSplit);
18// Initial
19checkactivateSplit();
How It Works
- Text Splitting: SplitType converts each character into a
<span>
element, allowing for precise animation targeting. - Animation Trigger: The JavaScript script adds the
.is-active
combo class to.split-anim
when the text scrolls into view. - Sequential Animations: CSS variables like
--j
,--i
and pseudo classes such as:nth-child
control the staggered animation effect for each character.
Advanced Customizations
Substitute SplitType with <Span>
For static or non-dynamic text content, manually wrapping characters, words, or lines in <span>
tags can be a lightweight and efficient alternative to using SplitType. This method eliminates the dependency on JavaScript, ensuring that your animations are not only faster but also accessible to users with JavaScript disabled.
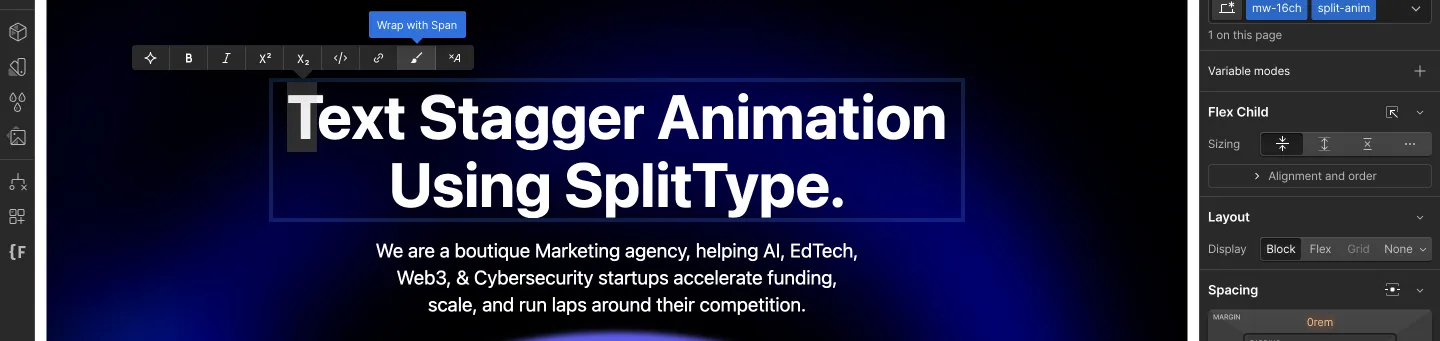
Why Choose <span>
Wrapping?
- Performance Boost: By directly coding the text structure in HTML, you bypass the runtime processing required by JavaScript libraries, reducing overhead.
- SEO and Accessibility: Search engines and assistive technologies can parse your content more reliably without relying on JavaScript execution.
- Fine-Grained Control: You have full control over the markup, allowing precise adjustments to animations or styling.
Limitations of <span>
Wrapping
- Time-Consuming: For larger blocks of text or frequently changing content, this method requires significant manual effort.
- Scalability Issues: If you need to update or manage the content dynamically, manual
<span>
wrapping becomes impractical with the Webflow's Rich Text Element. - Reusability: Unlike SplitType, which can automate the process for multiple elements, manual wrapping lacks flexibility across different parts of your project.
In conclusion, while substituting SplitType with <span>
tags can enhance performance and accessibility, it is best suited for static content with limited updates. For dynamic or large-scale projects, automated solutions like SplitType remain a more practical choice.
Speed and Timing
Adjust the speed and stagger timing by modifying the CSS animation properties:
1animation: split-anim 0.2s ease-out forwards; /* Adjust overall duration */
2animation-delay: calc(var(--j) * 0.3s + var(--i) * 0.07s); /* Adjust stagger */
Multi-Line Compatibility
SplitType supports splitting text into lines and words. Update the JavaScript initialization as needed:
1let text = new SplitType('.split-anim', { types: 'lines, words, chars' });
Interactive Animations
Combine this animation with hover states for dynamic effects. For example:
1.split-anim:hover .char{transform: rotate(-10deg) translateY(-20%);}
Benefits of using CSS and Vanilla JS
Using CSS and Vanilla JS for text animations offers significant advantages, especially when considering performance and accessibility. Here's why:
- Fallback for Non-JS Browsers: A key advantage of this approach is that the site's visitors will still experience a readable and functional design even if JavaScript is disabled in their browser. Since the initial states of the text are not hidden or set to be invisible, users can see the text in its static form without relying on JavaScript to render it.
- Efficient Performance: Vanilla JS and CSS animations are lightweight, reducing the need for heavy dependencies or additional libraries. This approach ensures faster page loading times and smoother animations, enhancing the overall user experience.
- Precision Control: By leveraging CSS variables and pseudo-classes, you have fine-grained control over staggered animations without the overhead of external libraries. This keeps your codebase clean and maintainable.
- Accessibility-Friendly: Animations initiated through a combo class (.is-active) ensure that only visible text is animated when it enters the viewport. This minimizes any potential disruptions for screen readers or assistive technologies, maintaining a focus on usability for all users.
- Reduced Development Overhead: This method eliminates the need to rely on third-party libraries, which can introduce complexities or compatibility issues. Instead, you achieve the desired effect using native web technologies, making debugging and customization simpler.
By adopting CSS and Vanilla JS for your text animations, you ensure a balanced approach that delivers impactful visuals without compromising accessibility or performance.
What are the Alternatives?
While using CSS and Vanilla JS for text animations is efficient and accessible, there are alternative methods that cater to different use cases or developer preferences. Here are some of the most notable options:
1. GSAP (GreenSock Animation Platform)
- Why Use It: GSAP is a powerful library for creating complex animations with precision. It supports advanced features like timeline control, easing, and multi-step animations.
- Drawbacks: GSAP adds additional weight to your project and may be unnecessary for simple text animations. It also requires JavaScript, meaning the animations won't render if JS is disabled.
When to Choose:
- You need intricate animations, such as morphing shapes, 3D effects, or chaining multiple animations across different elements.
- You need to speed up development, meaning thespeed to deliver is prioritized over performance.
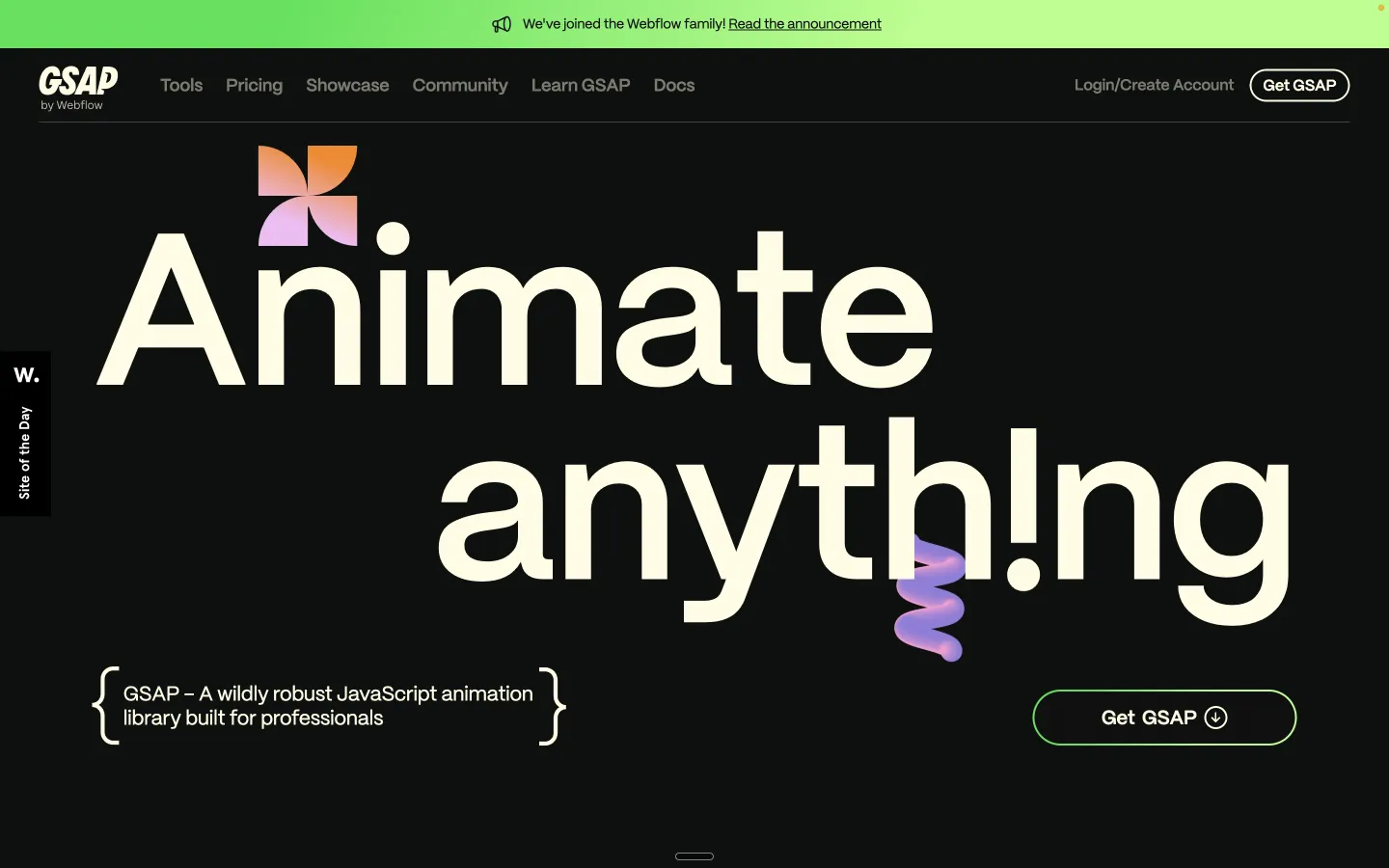
2. Anime.js
- Why Use It: Anime.js is a lightweight library that simplifies animations for various DOM elements, including text. It offers chainable animations, keyframes, and SVG support.
- Drawbacks: Similar to GSAP, it adds weight to the project and may complicate performance optimization for simpler effects.
When to Choose:
- Anime.js is suitable for projects requiring smooth, customizable animations but not the full complexity of GSAP.
- Your main objective is fast development of a limited set of animations.

3. Webflow Interactions
- Why Use It: Webflow’s built-in interactions allow you to create animations without writing code. It’s user-friendly and integrates seamlessly with other Webflow features.
- Drawbacks: Webflow interactions don’t offer the level of precision or customization possible with libraries like GSAP or Vanilla JS. Additionally, splitting text into characters or words isn’t natively supported. Lastly, all animations made in Webflow serve JS to a global file, meaning that even if you don't use it in certain pages, the code will still be there, hence bloated code base and worse performance.
When to Choose:
- Use Webflow interactions for basic animations when coding isn't an option or when working entirely within the Webflow ecosystem.
Overall, the best method depends on your specific needs. Do you need to deliver faster, or do you need to optimize the site's performance? At Tilipman Digital, we do our best to optimize for performance as much as possible and avoid using external libraries where they are not needed.
Think of using libraries as of using a fork instead of a toothpick to clean your teeth: it might work, but it’s overkill, less efficient, and risks breaking something in the process. Stick to the right tool for the job — precise, effective, and reliable.
Conclusion
SplitType makes it simple to craft engaging text animations that elevate your website's design. By leveraging CSS, JavaScript, and thoughtful animation timing, you can create a polished, professional effect that captures user attention. Whether you’re building a Webflow site or enhancing an existing one, these techniques will help you stand out.
If performance and accessibility are top priorities, however, implementing such animations can become a complex process. In such cases, careful optimization is required to balance aesthetics with usability. For those aiming to ensure their Webflow projects not only look stunning but also perform seamlessly, partnering up with a Webflow Development Agency that focuses on these issues can be a smart decision that save you long hours.